scp (secure copy) a file to the same path on a remote system
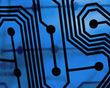
I've been copying files from one server to the symmetrical cluster partner a lot last week.
It's always
scp /path/to/directory/file remote:/path/to/directory/
That gets boring after a while but does not really warrant setting up a full blown config management solution like salt, puppet or ansible.
So here is scpover[1.5kB].
It reduces the effort to
scpover /path/to/directory/file
This will copy "file" from "/path/to/directory/" into exactly the same directory on the remote cluster partner. Which system to target is detected from the hostname of the local system and hard-coded into the script.
Scpover can also take multiple filepaths on one command line so you can beam over a few related config files from multiple locations in one go.
Not an atomic change but at least all within a reasonably short timespan.
- #!/bin/bash
- # scpover v0.1
- # Copyright (c) 2014 Daniel Lange, http://daniel-lange.com.
- # Released into the public domain. NO LIABILITY ACCEPTED WHATSOEVER. USE AT YOUR OWN RISK.
- READLINK="/bin/readlink"
- TARGET=""
- if [[ -v HOSTNAME ]] ; then
- case "${HOSTNAME,,}" in
- ashley)
- TARGET="marykate"
- ;;
- marykate)
- TARGET="ashley"
- ;;
- *)
- echo "Error: I have no cluster partner for ${HOSTNAME}. Exiting."
- exit 2
- ;;
- esac
- else
- echo "Error: I need \$HOSTNAME set to know where to copy."
- exit 1
- fi
- if [[ "$1" == "-h" || "$1" == "--help" ]] ; then
- echo "$0: <file1> [file2] ..."
- echo " Copy files to the cluster partner via scp into the same directory as where is resides on the source host."
- echo " Links (symbolic or hard) will be resolved and copied as a regular file. That's a feature/bug of scp."
- echo " The target is determined from a pre-defined list built into the program. So don't specify one."
- echo " HOSTNAME: ${HOSTNAME:-not set!}"
- exit 0
- fi
- if [[ ! -x $READLINK ]] ; then
- echo "ERROR: I need readlink to work. What strange system is this?"
- exit 3
- fi
- if [[ $# -lt 1 ]] ; then
- echo "I need at least one file to copy over to the cluster partner."
- exit 1
- fi
- echo "Copying from $HOSTNAME to $TARGET..."
- for f in "$@"; do
- FULLNAME=""
- FULLNAME=$($READLINK -n -f "$f")
- if [[ -f "$f" ]] ; then
- echo "${FULLNAME} -> ${TARGET}:${FULLNAME%/*}/"
- scp -p -C "$f" "${TARGET}:${FULLNAME%/*}/"
- else
- echo "Warning: $f ($FULLNAME) is not a regular file. Skipping."
- fi
- done
You need to define the cluster partner for each of your systems from line 12.
If you have larger clusters than pairs, think of deploying a config management solution.
And then make TARGET a list and add a loop around the echo and scp commands in lines 53f. .
Oh, and of course scpover can self-deploy:
root@marykate:/usr/local/bin# scpover scpover
Copying from marykate to ashley...
/usr/local/bin/scpover -> ashley:/usr/local/bin/
scpover 100% 1509 1.5KB/s 00:00
Comments
Display comments as Linear | Threaded
Ben on :
A cheeky alternative way to do it is
scp {,remote:}/path/to/file
. That way you also still have control over the local/remote part, at the cost of readabilityCharles on :
Thanks to Ben for the detailed explanation.