Encrypting files with openssl for synchronization across the Internet
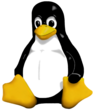
Well, shortly after I wrote about encrypting files with a keyfile / passphrase with gpg people asked about a solution with openssl.
You should prefer to use the gpg version linked above, but if you can't, below is a script offering the same functionality with openssl.
You basically call crypt_openssl <file> [<files...>]
to encrypt file
to file.aes
using the same keyfile as used in the gpg script (~/.gnupg/mykey001
per default).
A simple crypt_openssl -d <file.aes> [<files.aes...>]
will restore the original files from the encrypted AES256 version that you can safely transfer over the Internet even using insecure channels.
Please note that you should feed compressed data to crypt_openssl
whenever you can. So use preferably use it on .zip
or .tar.gz
files.
#!/bin/bash
# crypt_openssl v0.1
# Copyright (c) 2013 Daniel Lange, http://daniel-lange.com.
# Released into the public domain. NO LIABILITY ACCEPTED WHATSOEVER. USE AT YOUR OWN RISK.
# openssl builds a hash over the CRYPT_KEY keyfile (sha256 chosen below)
#
CRYPT_KEY="${HOME}/.gnupg/mykey001"
METHOD="enc -e"
if (($# == 0)) || [[ "$1" == "--help" ]] || [[ "$1" == "-h" ]]; then
echo "Usage: $0 [-d] <filename> [<filename> ...]"
exit 1
fi
if [[ ! -e "$CRYPT_KEY" ]]; then
echo "Error: Cryptographic key does not exist on this system."
exit 2
fi
if [[ "$1" == "--decrypt" ]] || [[ "$1" == "-d" ]]; then
METHOD="enc -d"
shift
fi
for INFILE in "$@"; do
if [[ "$METHOD" =~ " -e" ]] ; then
OUTFILE=$INFILE.aes
echo "Encrypting $INFILE to $OUTFILE..."
else
OUTFILE=${INFILE%.aes}
echo "Decrypting $INFILE to $OUTFILE..."
fi
cat "$CRYPT_KEY" | tr -d "\n\r\000" | openssl $METHOD -aes-256-cbc -pass fd:0 -md sha256 -v -in "$INFILE" -out "$OUTFILE"
done
Download crypt_openssl (1kB).
The file pointed to by CRYPT_KEY
is your keyfile, your shared secret, that you need to have available to be able to decode the .aes files again (e.g. on a different PC).
Please see the previous blog post to see how to create a CRYPT_KEY
keyfile securely.
Note:
- There is no limit on the
CRYPT_KEY
file size (as in the gpg variant) as openssl will just compute a hash over the file as a passphrase. - A file encrypted with crypt_gpg cannot be decoded with crypt_openssl and vice versa. Gpg and openssl use slightly different file formats.
Comments
Display comments as Linear | Threaded