zip2dir (expand a zip to a directory of the same name)
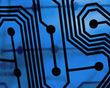
I needed to expand a lot of jars (Java zips) and other zips of various names into directories of the same name for each file. With 6,239 files of which some are jars, some other zips and many xml and other filetypes all not properly identified by a file extension, this gets a bit too much to do manually.
So:
Finding candidates for these is easy with find . -type f
.
The file is most probably a zip archive if the first two characters are "PK", good old Phil Katz' signature. A friendly head -c 2
checks that.
All combined with some rudimentary error checking:
- #!/bin/bash
- # There is little data security here, so know what you're doing.
- # All risks in using this code are yours. It moves and deletes files quite stupidly.
- # (c) Daniel Lange, 2009, v0.01, released into the public domain
- if [ $# -ne 1 ] ; then
- echo "Error: $0 expects exactly one argument, a (fully qualified) path/to/a/zipfile"
- exit 1
- fi
- if [ ! -r $1 ] ; then
- echo "Error: file does not exist or no read permission on $1"
- exit 2
- fi
- if [ ! -w "$(dirname $1)" ] ; then
- echo "Error: cannot write to directory of $1"
- exit 3
- fi
- if [ "$(head -c 2 $1)" == "PK" ] ; then
- mv $1 $1.tmp
- mkdir -p $1
- unzip -d $1 $1.tmp
- rm $1.tmp
- else echo "$1 is not a zipfile"
- fi
Download available here (1KB).
Typical usage:
This will expand all zips under the current directory.
Leave the zip2dir
out for a dry run (xargs will just print to the tty then). Look at the -exec
switch when digging around a bit more into what find
can do for you.